Discord Flows
Discord Integration for [Flows.network](https://test.flows.network)
Quick Start
There is a echo bot, but plain text:
```rust
use discordflows::{getclient, listentoevent, model::Message};
[no_mangle]
[tokio::main(flavor = "current_thread")]
pub async fn run() {
let token = std::env::var("DISCORD_TOKEN").unwrap();
listen_to_event(token.clone(), move |msg| handle(msg, token)).await;
}
async fn handle(msg: Message, token: String) {
let client = getclient(token);
let channelid = msg.channel_id;
let content = msg.content;
if msg.author.bot {
return;
}
_ = client
.send_message(
channel_id.into(),
&serde_json::json!({
"content": content,
}),
)
.await;
}
```
[get_client()] is a Discord
constructor that represents a bot.
If you don't have a token, please see this section.
[listentoevent()] is responsible for registering a listener for the bot
represented by the bot_token
. When a new Message
coming, the callback
is called with received Message
.
Creating a Bot Account
The following is excerpted from
discord.py docs
- Make sure you’re logged on to the Discord website.
- Navigate to the application page.
- Click on the “New Application” button.
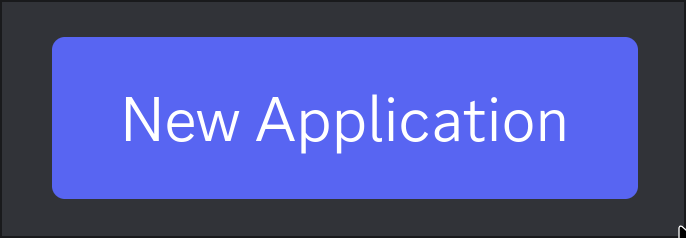
- Give the application a name and click “Create”.
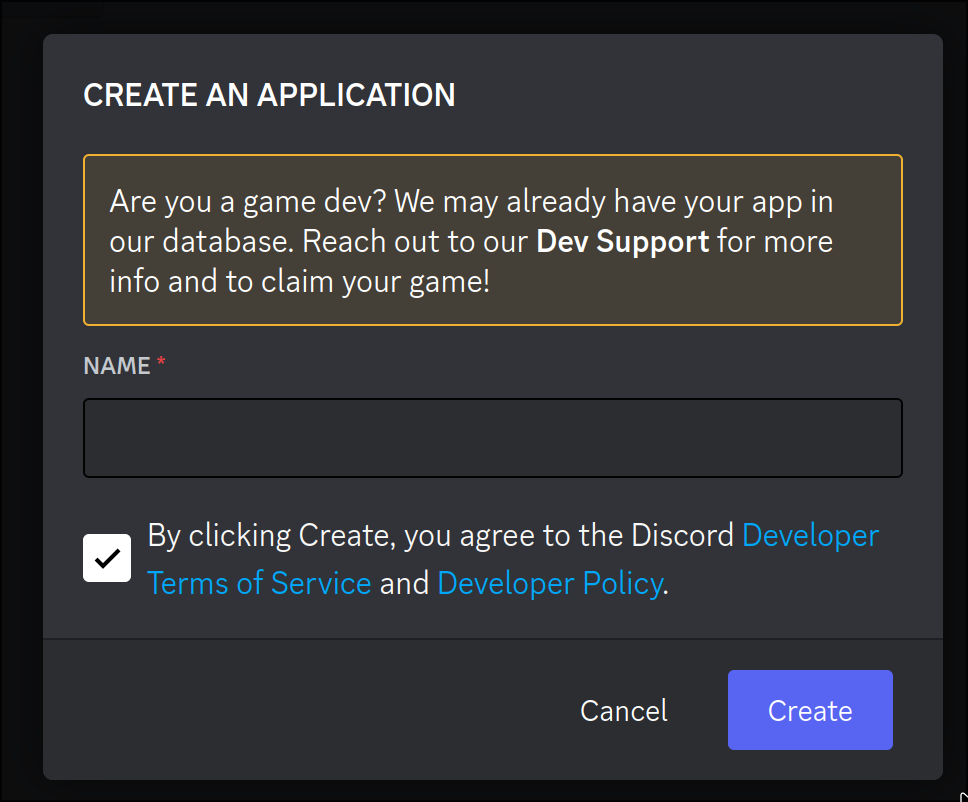
- Navigate to the “Bot”.
- Make sure that Public Bot is ticked if you want others to invite your bot.
- Click on the "Reset Token" button.
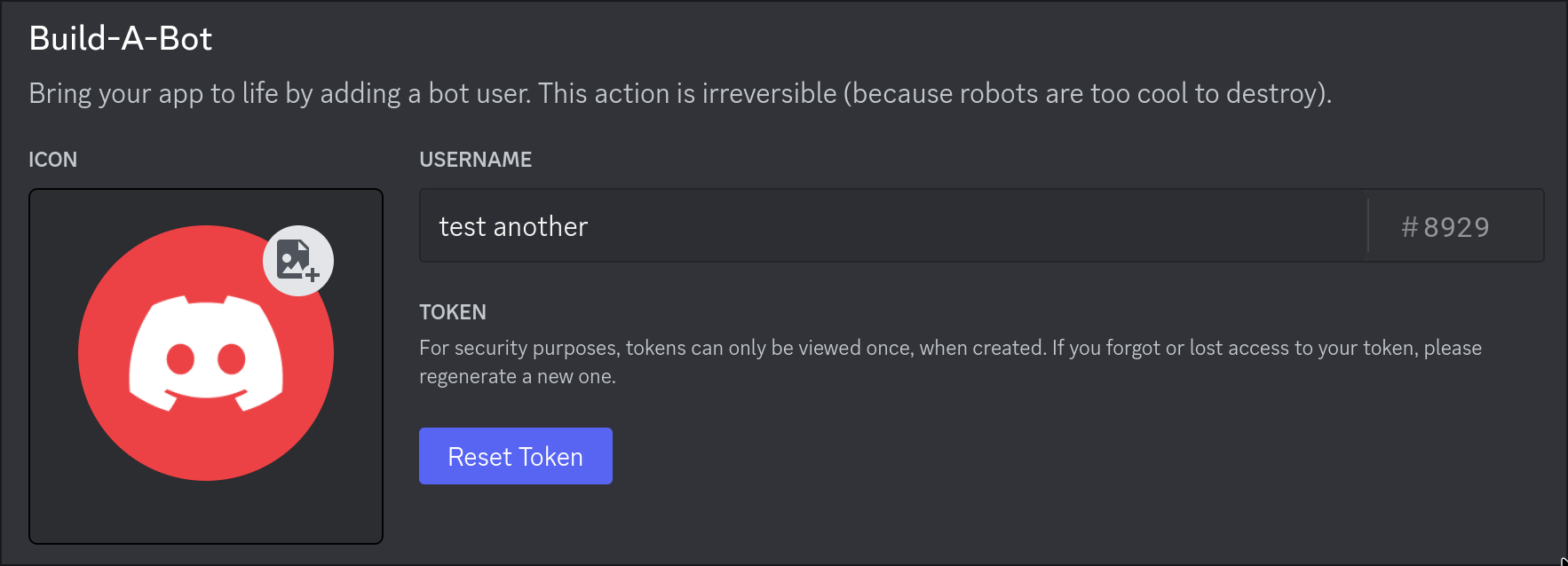
- Confirm reset by clicking "Yes, do it!" button.
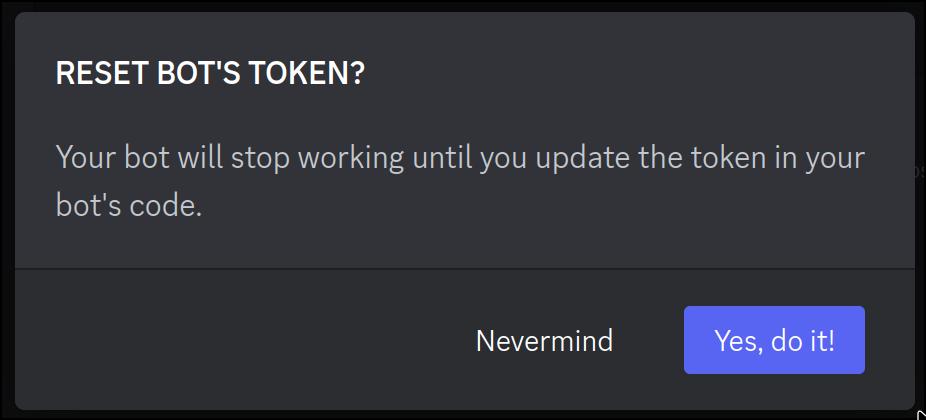
- Copy the token using the “Copy” button.
> It should be worth noting that this token is essentially your bot’s password.
> You should never share this with someone else.
> In doing so, someone can log in to your bot and do malicious things,
> such as leaving servers, ban all members inside a server,
> or pinging everyone maliciously.
>
> The possibilities are endless, so do not share this token.
>
> If you accidentally leaked your token,
> click the “Regenerate” button as soon as possible.
> This revokes your old token and re-generates a new one.
> Now you need to use the new token to login.
- Keep your token in a safe place, the token will only be shown once.
Inviting Your Bot
So you’ve made a Bot User but it’s not actually in any server.
If you want to invite your bot you must create an invite URL for it.
- Make sure you’re logged on to the Discord website.
- Navigate to the application page
- Click on your bot’s page.
- Go to the “OAuth2” tab.
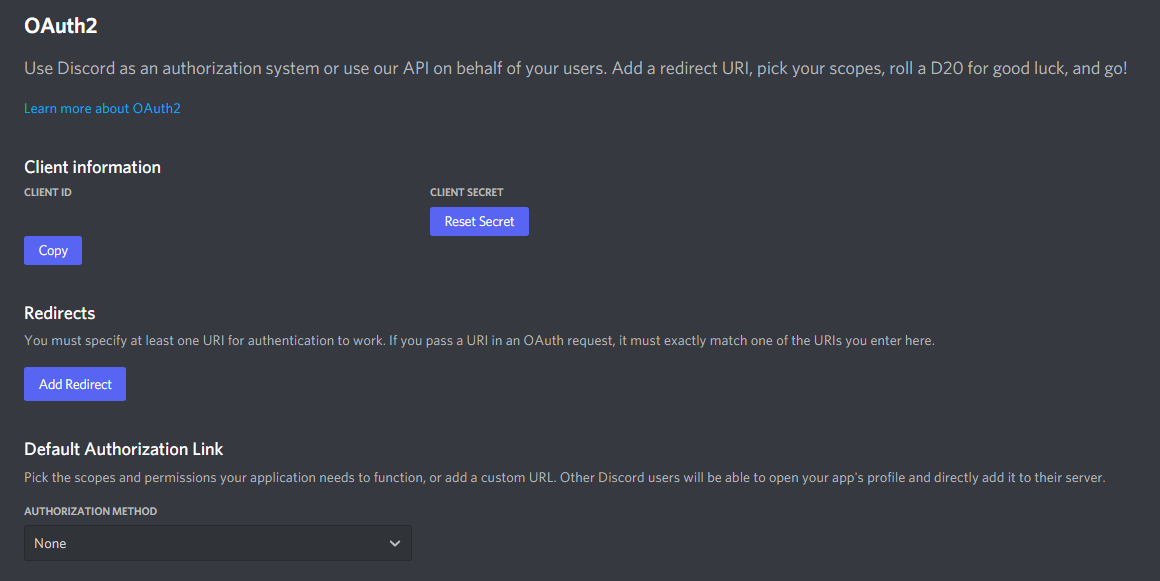
- Tick the “bot” checkbox under “scopes”.
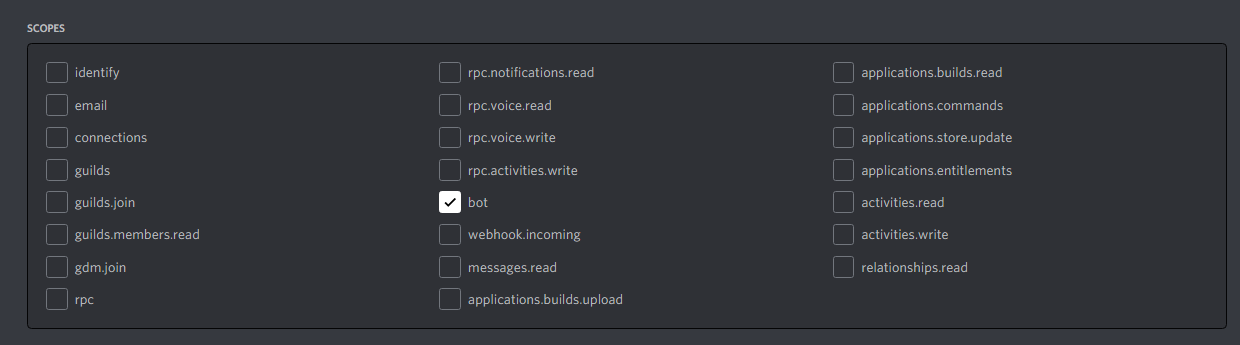
- Tick the permissions required for your bot to function under “Bot Permissions”.
- Please be aware of the consequences of requiring your bot to have the “Administrator” permission.
- Bot owners must have 2FA enabled for certain actions and permissions when added in servers that have Server-Wide 2FA enabled. Check the 2FA support page for more information.
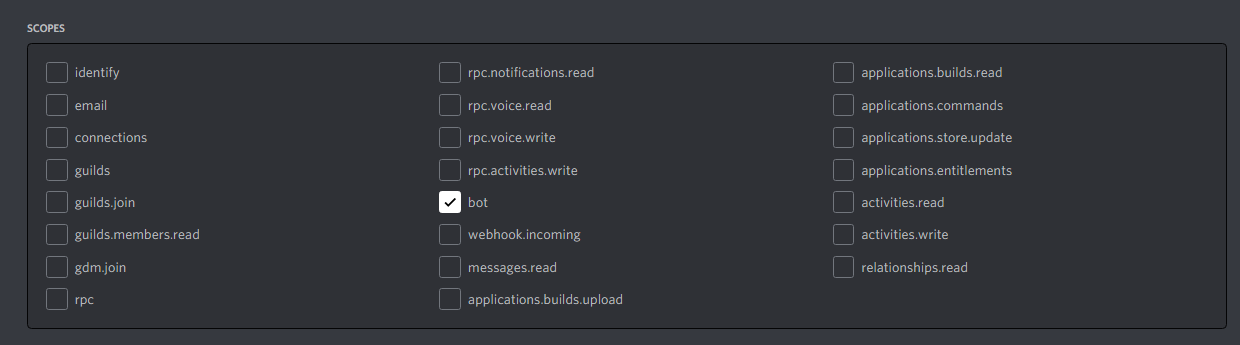
- Now the resulting URL can be used to add your bot to a server. Copy and paste the URL into your browser, choose a server to invite the bot to, and click “Authorize”.
The person adding the bot needs “Manage Server” permissions to do so.